Cart and product integration
Full cart and product integration enables your shoppers to add to cart from within the player and allows you to enrich your products with variants (e.g. color and size), stock availability, prices, and more.
Without integration (default):
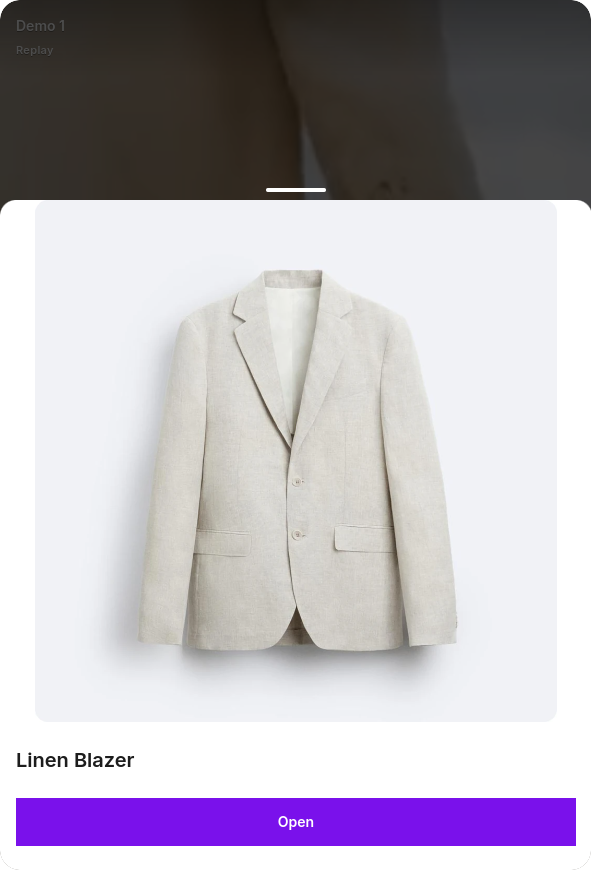
With integration:
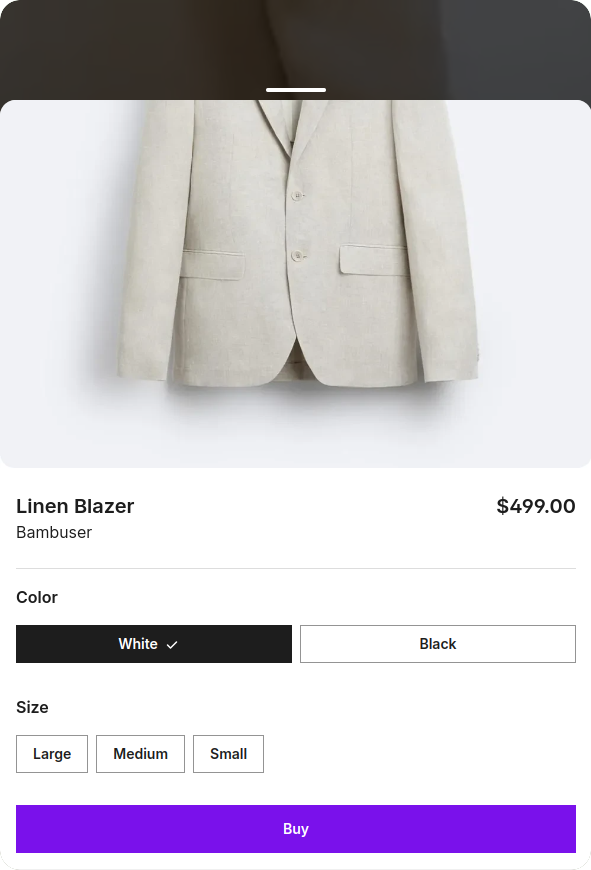
An integration consists of a JavaScript function that lives on your website which the player calls to request information and inform your website about events taking place inside the player (such as when a user adds to cart). This integration function needs to exist on all pages on your website where a Bambuser player appears.
// Integration code (on your website)
window.onBambuserLiveShoppingReady = player => {
player.configure({
currency: 'USD',
locale: 'en-US',
});
// React to instructions sent from the player
player.on(eventName, event => {
// Your application logic
});
};
Full working example
// Integration code (on your website)
window.onBambuserLiveShoppingReady = (player) => {
player.configure({
locale: 'en-US',
currency: 'USD',
});
// React to instructions sent from the player
player.on(player.EVENT.ADD_TO_CART, () => {});
player.on(player.EVENT.UPDATE_ITEM_IN_CART, () => {});
player.on(player.EVENT.CHECKOUT, () => {});
player.on(player.EVENT.PROVIDE_PRODUCT_DATA, (event) => {
event.products.forEach(({ ref: sku, id: productId }) => {
player.updateProduct(productId, (factory) => factory
.locale('en-US')
.currency('USD')
.product((p) => p
.name('Linen Blazer')
.brandName('Bambuser')
.sku('111')
.variations((v) => [
v()
.sku('1111')
.name('White')
.imageUrls(['https://demo.bambuser.shop/wp-content/uploads/2024/07/Blazer-Beige-1.png'])
.attributes((a) => a
.colorName('White')
.colorHexCode('#FFF'),
)
.sizes((s) => [
s()
.price((pr) => pr.current(499).currency('USD'))
.name('Small')
.sku('11111'),
s()
.price((pr) => pr.current(499).currency('USD'))
.name('Medium')
.sku('11112'),
s()
.price((pr) => pr.current(499).currency('USD'))
.name('Large')
.sku('11113'),
]),
v()
.sku('1112')
.name('Black')
.imageUrls(['https://demo.bambuser.shop/wp-content/uploads/2024/07/Blazer-Black.png'])
.attributes((a) => a
.colorName('Black')
.colorHexCode('#000'),
)
.sizes((s) => [
s()
.price((pr) => pr.current(499).currency('USD'))
.name('Small')
.sku('11121'),
s()
.price((pr) => pr.current(499).currency('USD'))
.name('Medium')
.sku('11122'),
s()
.price((pr) => pr.current(499).currency('USD'))
.name('Large')
.sku('11123'),
]),
]),
)
);
});
});
};
If you already have Cart Integration for Live Player implemented on your website you already have what you need. Shoppable Video will use the existing integration if available.
Implementation
Prerequisites
- Your integration function must be able to fetch data from your product portfolio.
- Your integration function must be able to update the cart on your website.
- Your videos must have products added to them on your workspace.
Building the onBambuserLiveShoppingReady
function
The player will look for a function called onBambuserLiveShoppingReady
on the global window
object and call it with a reference to the player itself. The player
reference provides an interface for your code to interact with the player. The basic outline for the main function looks like this:
window.onBambuserLiveShoppingReady = player => {
// This function will be called when a player is fully loaded.
// If you have multiple players it will be called once per player.
// Use the "player" object to interact with the player.
};
In the main function you need to configure the locale and currency the player should use:
window.onBambuserLiveShoppingReady = player => {
// This function will be called when a player is fully loaded.
// If you have multiple players it will be called once per player.
// Use the "player" object to interact with the player.
// Configure the player
player.configure({
currency: 'USD',
locale: 'en-US',
});
};
Then, you need to implement handling of 4 specific instructions the player may pass to your code:
Requirement: All 4 event handlers listed below must be defined.
window.onBambuserLiveShoppingReady = player => {
// This function will be called when a player is fully loaded.
// If you have multiple players it will be called once per player.
// Use the "player" object to interact with the player.
// Configure the player
// ... see above ...
player.on(player.EVENT.ADD_TO_CART, (event, callback) => {
// Handle event: User added items to cart in the player
});
player.on(player.EVENT.UPDATE_ITEM_IN_CART, (event, callback) => {
// Handle event: User changed the number of items to cart in the player
});
player.on(player.EVENT.CHECKOUT, () => {
// Handle event: User clicked on the checkout button
});
player.on(player.EVENT.PROVIDE_PRODUCT_DATA, event => {
// Handle events: Player requests product data
});
};
See the following pages for how the event handlers should be implemented:
Troubleshooting
Cart Integration FAQ
Bambuser Live’s in-player Product Detail Page (PDP) currently supports a maximum of two variations (e.g., Color and Size). This means the player can display up to two dropdowns for product selection.
If you have a third variation (e.g., Material), you will need to either:
- Combine it with one of your other variations (e.g., merge Material with Size or Color).
- Opt not to use Cart Integration for these products.
Every time you open a new instance of the Bambuser Player, the cart will be empty. This is by design. The bi-directional cart sync is currently not supported.
To display both prices, use the PROVIDE_PRODUCT_DATA
event handler and include .current()
and .original()
in the .price()
method:
.current(size.current)
sets the discounted price (e.g., 50)..original(size.original)
sets the original price (e.g., 75).
If current price is lower than original price, the player will display both if provided (e.g., "$50 (was $75)"). Ensure your product data includes both prices.